Poker Bot Tournament
Open to all current (and post!) graduate students of the Drexel Physics Department
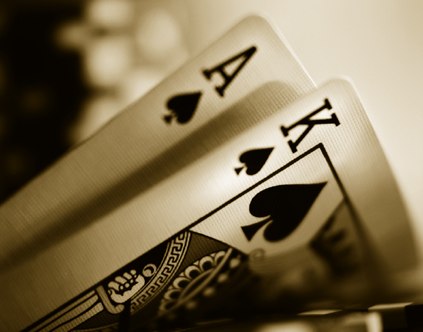
Something about poker seems to attract physicists. It may be the mathematical finesse required to compute odds or perhaps the parallels to uncertainty to we find in other branches of physics. Either way, the game represents a unique, hard (yet finite!) open problem. Thus, we intend to solve it. In our spare time. I present to you the first Drexel Physics (bot) Tournament. As humans are fallible, your entry will be in the form of a program.
Rules
You will submit a program to play heads-up Texas Hold'em. Your program will play every other program in a series (1000) of tournaments. A point is awarded for the winner of the series. The program with the most points after each set is conducted will be declared the winner. Each player will start with 1000 chips. The big blind structure for a single tournament is listed below. If any tournament continues for longer than 1000 hands, the tournament will be considered a draw (realistically this is only possible if each player folds every hand). Every twenty hands the blinds will raise to the following amount. B = [2,4,8,16,32,50,75,100]
If two players elect to play a strategy of "CALL" every hand, each game reduces to a coin flip. Empirically, this gives an expected number of hands:

Your code must also run within an "acceptable" time frame. In general your code should spend no longer then one second per hand.
Source code
Dealer code (requires Python 2.5):PBOT.py
GAMEPLAY.py
DECK.py
combinations.py
p1.py
p2.py
To run copy all files to a local directory. To play against yourself:
python PBOT.py
I've created two sample programs to get you started. To test yourself against a program that will go all-in if they hold an Ace:
python PBOT.py p1.py
The other program is similar, it goes all-in if it's pocket cards are paired:
python PBOT.py p2.py
To play these two computer programs against themselves:
python PBOT.py p1.py p2.py
The program itself accepts an (A) as an all-in bet, (C) as a call or check and any valid bet. Valid bets are either the amount owed to the pot or at least the min raise. All bets made greater then the players stack will be considered an all-in. All bets that are less than the amount owed will be considered a fold. A bet in the range of the amount owed to the min raise will be considered a CALL and the player will put in the amount owed to the table. Any other input (negative, decimal, or alpha) will be considered to be a FOLD. To keep a presistant state (and for speed reasons) your program will have the information fed to it. This is done by piping Standard IN and Standard OUT to your program. The dealer will wait once the command "MOVE" is sent. For an example see p1.py as a template.
Along with the "MOVE" tag, your code will be given information and action by the players. As an example of some of the input:
INFO NAME p1.py
INFO ONAME Jack
INFO STACK 0
INFO OSTACK 0
INFO POT 2000
INFO MINRAISE 1988
INFO OWE 10
INFO HOLE 9d Ad
INFO FLOP
INFO TURN
INFO RIVER
ACTION Player p1.py bets: 994
ACTION Player Jack bets: 1003
MOVE
ACTION Player p1.py bets: 0
ACTION Player p1.py wins: 2000
INFO p1.py 9d Ad Flush [[1, [12, 11, 7, 3, 2]]] 2000
INFO Jack 2s Qc Pair [[2, [0]], [1, [11, 10, 4]]] 0
INFO GAMEOVER 4
END
Submission
The contest will be open till the end of August (31st). All submissions should be sent to hoppe at drexel.edu with the DREXEL POKER BOT in the title. The code posted above will be the code used to judge the programs. All updates to the code and the contest will be posted below.If you have a friend outside the department who wishes to enter, they may do so as long as they have a sponsor within physics graduate student group. Multiple entries are allowed but your programs will not play themselves to determine score (and will be weighted accordingly).
Updates
Aug 4A bug was fixed in GAMEPLAY.py. If two players went all-in with unequal stacks, both players would push their entire stack into the pot. The correct action is for the larger stack player to push only an amount equal to the smaller stack. This is now fixed.
All bets that are somewhere from the amount owed to the min raise are now considered a CALL rather then an illegal bet and FOLD. Bets below the amount owed are still considered an illegal bet and hence a FOLD.